How to inject mock abstract class of Technology
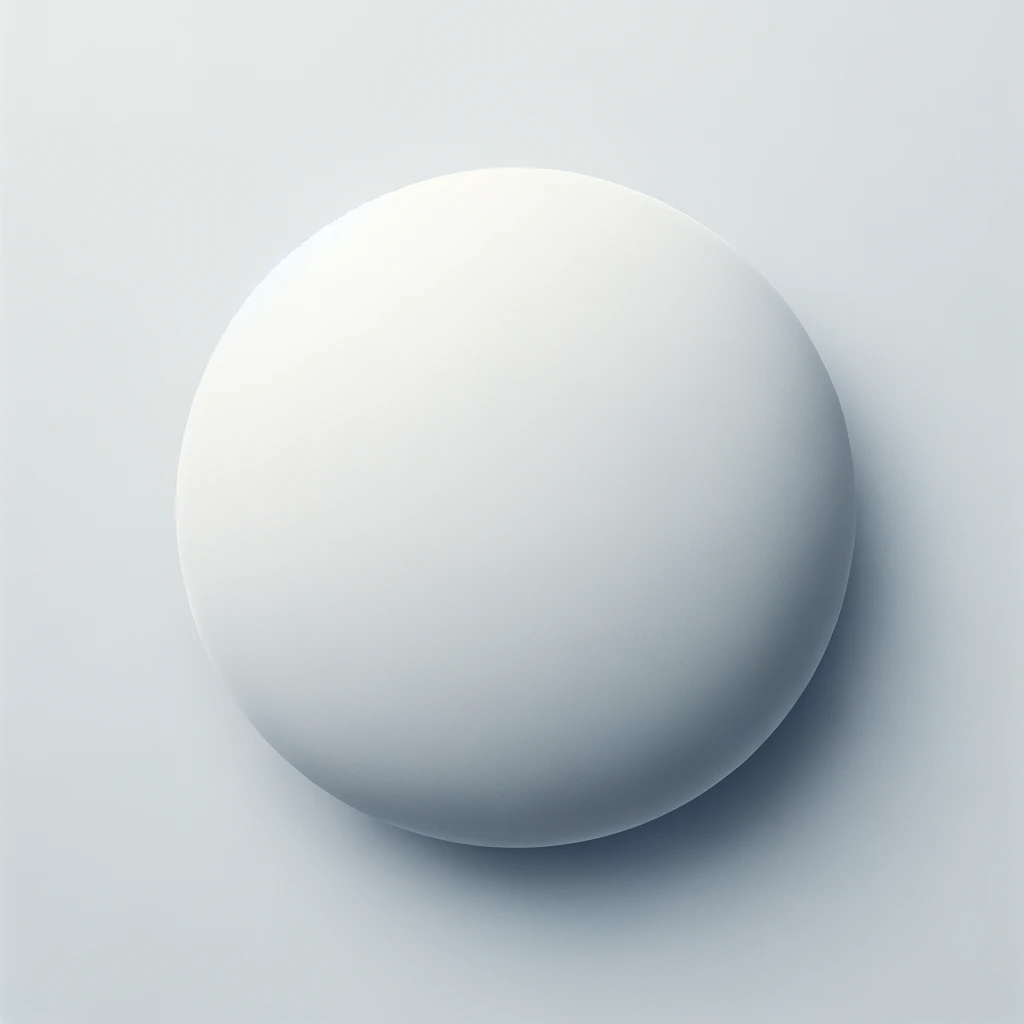
In my BotController class I'm using the Gpio class to construct distinct instances of Gpio: But with typescript, if you inject a class into a constructor (and I assume methods), you don't get the class constructor, you get an instance of the class. To inject a constructor instead of an instance, you need to use typeof: Because according to the ...1 Answer. It doesn't work like this. You should create an mock of the Interface and inject this mock implementation into class under test: public interface Foo { String getSomething (); } public class SampleClass { private final Foo foo; public SampleClass (Foo foo) { this.foo = foo; } }17. As I know, field injection is not recommended. Should use constructor instead. What I'm trying to do here is using @Autowired in the constructor of the base class, and make it accessible for all the subclasses. In some subclasses, I also need some specific beans to be @Autowired from their constructors.Mockito Get started with Spring 5 and Spring Boot 2, through the Learn Spring course: >> CHECK OUT THE COURSE 1. Overview In this tutorial, we'll analyze various use cases and possible alternative solutions to unit-testing of abstract classes with non-abstract methods.0. I think the following code achieves what you want. Creating a Mock from a CustomerController allows the setup the virtual method GetAge while still being able to use the GetCustomerDetails method from the CustomerController class. [TestClass] public class CustomerControllerTest { private readonly Mock<CustomerController> …builds a regular mock by passing the class as parameter: mockkObject: turns an object into an object mock, or clears it if was already transformed: unmockkObject: turns an object mock back into a regular object: mockkStatic: makes a static mock out of a class, or clears it if it was already transformed: unmockkStatic: turns a static mock back ...Apr 11, 2023 · We’ll apply @Autowired to an abstract class and focus on the important points we should consider. 2. Setter Injection. When we use @Autowired on a setter method, we should use the final keyword so that the subclass can’t override the setter method. Otherwise, the annotation won’t work as we expect. 3. Mockito mocking framework provides different ways to mock a class. Let’s look at different methods through which we can mock a class and stub its behaviors. Mockito mock method. We can use Mockito class mock() method to create a mock object of a given class or interface. This is the simplest way to mock an object.The new method that makes mocking object constructions possible is Mockito.mockConstruction (). This method takes a non-abstract Java class that constructions we're about to mock as a first argument. In the example above, we use an overloaded version of mockConstruction () to pass a MockInitializer as a second argument.Then we request that Nest inject the provider into our controller class: ... You want to override a class with a mock version for testing. Nest allows you ...Sep 7, 2021 · Currently, the unit test that I have uses mocker to mock each class method, including init method. I could use a dependency injection approach, i.e. create an interface for the internal deserializer and proxy interface and add these interfaces to the constructor of the class under test. I am mocking an abstract class like below: myAbstractClass = Mockito.mock(MyAbstractClass.class, Mockito.CALLS_REAL_METHODS); the problem …A MockSettings object is instantiated by a factory method: MockSettings customSettings = withSettings ().defaultAnswer ( new CustomAnswer ()); We’ll use that setting object in the creation of a new mock: MyList listMock = mock (MyList.class, customSettings); Similar to the preceding section, we’ll invoke the add method of a MyList instance ...May 5, 2015 at 15:30. Add a comment. 0. You cannot mock abstract classes, you have to mock a concrete one and pass that along. Just as regular code can't instantiate abstract classes. Share.29 thg 7, 2020 ... With JUnit, you can write a test class for any source class in your Java project. Even abstract classes, which, as you know, can't be ...Sep 3, 2020 · Now, in my module, I am trying to inject the service as : providers: [ { provide: abstractDatService, useClass: impl1 }, { provide: abstractDatService, useClass: impl2 } ] In this case, when I try to get the entities they return me the entities from impl2 class only and not of impl1 Aug 24, 2020 · Use xUnit and Moq to create a unit test method in C#. Open the file UnitTest1.cs and rename the UnitTest1 class to UnitTestForStaticMethodsDemo. The UnitTest1.cs files would automatically be ... Here is what I did to test an angular pipe SafePipe that was using a built in abstract class DomSanitizer. // 1. Import the pipe/component to be tested import { SafePipe } from './safe.pipe'; // 2. Import the abstract class import { DomSanitizer } from '@angular/platform-browser'; // 3. Important step - create a mock class which extends // from ...Cover abstract class method with tests in Jest. I have generic service class which is abstract. export default abstract class GenericService<Type> implements CrudService<Type> { private readonly modifiedUrl: URL; public constructor (url: string) { this.modifiedUrl = new URL (url, window.location.href); } public async get (path?: string, filter?:1 Answer. Sorted by: 1. If you want to use a mocked logger in the constructor, you it requires two steps: Create the mock in your test code. Pass it to your production code, e.g. as a constructor parameter. A sample test could look like this:Implement abstract test case with various tests that use interface. Declare abstract protected method that returns concrete instance. Now inherit this abstract class as many times as you need for each implementation of your interface and implement the mentioned factory method accordingly. You can add more specific tests here as well. Use test ...In order to be able to mock the Add method we can inject an abstraction. Instead of injecting an interface, we can inject a Func<int, int, long> or a delegate. Either work, but I prefer a delegate because we can give it a name that says what it's for and distinguishes it from other functions with the same signature. Here's the delegate and …Mar 23, 2019 · I'm writing the Junit test case for a class which is extended by an abstract class. This base abstract class has an autowired object of a different class which is being used in the class I'm testing. I'm trying to mock in the subclass, but the mocked object is throwing a NullPointerException. Example: [TestMethod] public void ClassA_Add_TestSomething() { var classA = new A(); var mock = new Mock<B>(); classA.Add(mock.Object); // Assertion } I receive the following exception. Test method TestSomething threw exception: System.ArgumentException: Type to mock must be an interface or an abstract or non …4. Two ways to solve this: 1) You need to use MockitoAnnotations.initMocks (this) in the @Before method in your parent class. The following works for me: public abstract class Parent { @Mock Message message; @Before public void initMocks () { MockitoAnnotations.initMocks (this); } } public class MyTest extends Parent { @InjectMocks MyService ...Jun 24, 2020 · There are two ways to unit test a class hierarchy and an abstract class: Using a test class per each production class. Using a test class per concrete production class. Choose the test class per concrete production class approach; don’t unit test abstract classes directly. Abstract classes are implementation details, similar to private ... ColumnNames is a property of type List<String> so when you are setting up you need to pass a List<String> in the Returns call as an argument (or a func which return a List<String>) But with this line you are trying to return just a string. input.SetupGet (x => x.ColumnNames).Returns (temp [0]); which is causing the exception.Instead of doing @inject mock on abstract class create a spy and create a anonymous implementation in the test class itself and use that to test your abstract class.Better not to do that as there should not be any public method on with you can do unit test.Keep it protected and call those method from implemented classes and test only those classes. @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration({ "classpath:test-context.xml" }) public class MyTest { I would like to mock a value for my "defaultUrl" field. Note that I don't want to mock values for the other fields — I'd like to keep those as they are, only the "defaultUrl" field.1. Introduction In this quick tutorial, we’ll explain how to use the @Autowired annotation in abstract classes. We’ll apply @Autowired to an abstract class and focus on the important points we should consider. 2. Setter Injection We can use @Autowired on a setter method:Also consider constructor injection as opposed to field injection. It is preferred for this exact case; it is much easier to unit test when using constructor injection. You can mock all the dependencies and just instantiate the class to test, passing in all the mocks. Or even use setter injection.With JMockit, we can use the MockUp API to alter the real implementation of protected methods. All following examples will be done for the following class and we’ll suppose that are run on a test class with the same configuration as the first one (to avoid repeating code): public class AdvancedCollaborator { int i; private int privateField ...If you want to mock methods on an abstract class like this, then you need to make it either virtual, or abstract. As a workaround you can use not the method itself but create virtual wrapper method instead. public abstract class TestAb { protected virtual void PrintReal () { Console.WriteLine ("method has been called"); } public void Print ...Now I need to test the GetAllTypes methods in my controller class. My Test Class is below mentioned: using moq; [TestClass] public Class OwnerTest { public OwnerTest () { var mockIcomrepo = new Mock<IComRepository> (); var mockDbcontext = new Mock<Dbcontext> (); OwnerController owner = new OwnerController …For spying or mocking the abstract classes, we need to add the following Maven dependencies: JUnit Mockito PowerMock All the required dependencies of the project are given below: <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency> <dependency> <groupId>org.mockito</groupId>Basically, you probably want to pull out the logic in the abstract class to a behavior-object and use composition not inheritance. In all my years writing Typescript, I've used an abstract class exactly once. I used them a bit more in C#, but even there, they were are rarity. Step Two: If you really want an abstract class, test all the concrete ...The PHPUnit method getMockForAbstractClass() can be used to generate a partial mock where only the abstract methods of a given class are overridden. The argument list for getMockForAbstractClass() is similar to the argument list for getMock().The big difference is that the list of methods to mock is moved from being the second parameter to being the …39. The (simplest) solution that worked for me. @InjectMocks private MySpy spy = Mockito.spy (new MySpy ()); No need for MockitoAnnotations.initMocks (this) in this case, as long as test class is annotated with @RunWith (MockitoJUnitRunner.class). Share. With JMockit, we can use the MockUp API to aJul 3, 2020 · MockitoJUnitRunner makes the procesI'm using Mockito 1.9.5 to do some unit testin
Health Tips for R green bay packers
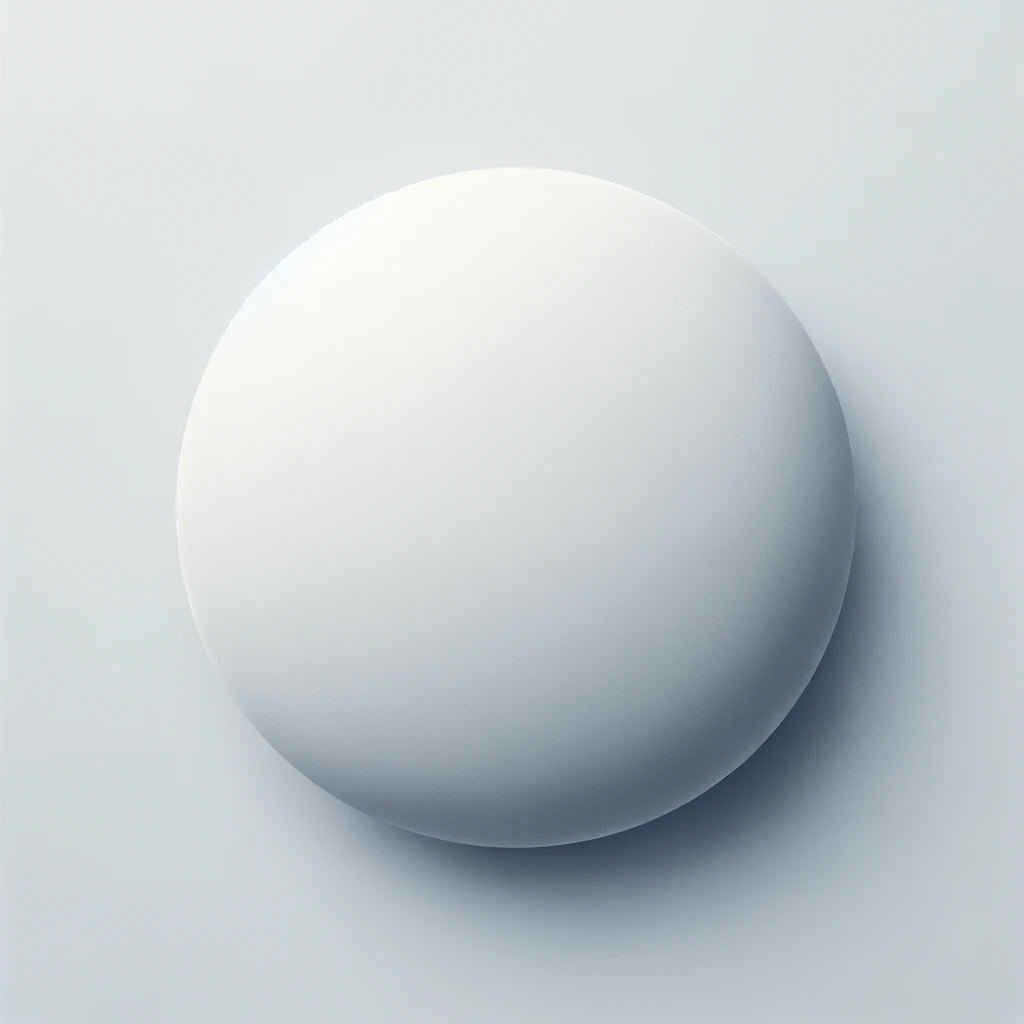
I want to test a class that calls an object (static method call in java) but I'm not able to mock this object to avoid real method to be executed. object Foo { fun bar() { //Calls third party sdk here } }Injecting a mock is a clean way to introduce such isolation. 2. Maven Dependencies. We need the following Maven dependencies for the unit tests and mock objects: We decided to use Spring Boot for this example, but classic Spring will also work fine. 3.The extension will initialize the @Mock and @InjectMocks annotated fields. with the @ExtendWith(MockitoExtension.class) inplace Mockito will initialize the @Mock and @InjectMocks annotated fields for us. The controller class uses field injection for the repository field. Mockito will do the same. Mockito can also do constructor and field …2. You can mock any method using when ().thenReturn () construct. Example: MyClass mc = Mockito.spy (new MyClass ("a","b","c")); when (mc.getStringFromExternalSource ()).thenReturn ("I got it from there!!"); So whenever the method 'getStringFromExternalSource ()' is invoked for the mocked object mc then it will return "I …The new method that makes mocking object constructions possible is Mockito.mockConstruction (). This method takes a non-abstract Java class that constructions we're about to mock as a first argument. In the example above, we use an overloaded version of mockConstruction () to pass a MockInitializer as a second argument.While it’s best to use a system like dependency injection to avoid this, MockK makes it possible to control constructors and make them return a mocked instance. The mockkConstructor (T::class) function takes in a class reference. Once used, every constructor of that type will start returning a singleton that can be mocked.I am trying to write some tests for it but cannot find any information about testing abstract classes in the Jasmine docs. import { Page } from '../models/index'; import { Observable } from 'rxjs/Observable'; export abstract class ILayoutGeneratorService { abstract generateTemplate (page: Page, deviceType: string ): Observable<string>; } …A MockSettings object is instantiated by a factory method: MockSettings customSettings = withSettings ().defaultAnswer ( new CustomAnswer ()); We’ll use that setting object in the creation of a new mock: MyList listMock = mock (MyList.class, customSettings); Similar to the preceding section, we’ll invoke the add method of a …Google Mock can mock non-virtual functions to be used in what we call hi-perf dependency injection. In this case, ... a free function (i.e. a C-style function or a static method). You just need to rewrite your code to use an interface (abstract class). Instead of calling a free function (say ... When you define the mock class using Google Mock ...1. You need to tell Rhino.Mocks to call back to the original implementation instead of doing its default behavior of just intercepting the call: var mock = MockRepository.GenerateMock<YourClass> (); mock.Setup (m => m.Foo ()).CallOriginalMethod (OriginalCallOptions.NoExpectation); Now you can call the Foo () …Previously, spying was only possible on instances of objects. New API makes it possible to use constructor when creating an instance of the mock. This is particularly useful for mocking abstract classes because the user is no longer required to provide an instance of the abstract class.Manual mock that is another ES6 class If you define an ES6 class using the same filename as the mocked class in the __mocks__ folder, it will serve as the mock. This class will be used in place of the real class. This allows you to inject a test implementation for the class, but does not provide a way to spy on calls.Mar 21, 2018 · You can use the abc module to write abstract classes in Python, but depending on which tool you use to check for unimplemented members, you may have to re-declare the abstract members of your ... I know of no way to inject a mock into a mock. What you could do with the SomeService mock is to mock the getter to always returnt he SomeClient mock. This would, however, require that within SomeService, someClient is only accessed through the getter. --- I would question the notion to test an abstract class and rather opt to provide …Mocking ES6 class imports. I'd like to mock my ES6 class imports within my test files. If the class being mocked has multiple consumers, it may make sense to move the mock into __mocks__, so that all the tests can share the mock, but until then I'd like to keep the mock in the test file. Jest.mock() jest.mock() can mock imported modules. When ...... class}) @ActiveProfiles("dev") public abstract class AbstractIntegrationTest { } ... Inject the mock request or session into your test instance and prepare your ...Jan 15, 2018 · and mock the UserService as well and assign it to the subject under test. Configure the desired/mocked behavior for the test. public class UserResourceTest { @Test public void test () { //Arrange boolean expected = true; DbResponse mockResponse = mock (DbResponse.class); when (mockResponse.isSuccess).thenReturn (expected); User user = mock ... Injecting a mock is a clean way to introduce such isolation. 2. Maven Dependencies. We need the following Maven dependencies for the unit tests and mock objects: We decided to use Spring Boot for this example, but classic Spring will also work fine. 3.Now I need to test the GetAllTypes methods in my controller class. My Test Class is below mentioned: using moq; [TestClass] public Class OwnerTest { public OwnerTest () { var mockIcomrepo = new Mock<IComRepository> (); var mockDbcontext = new Mock<Dbcontext> (); OwnerController owner = new OwnerController …Injecting Mockito Mocks into Spring Beans This article will show how to use dependency injection to insert Mockito mocks into Spring Beans for unit testing. Read more → 2. Enable Mockito Annotations Before we go further, let's explore different ways to enable the use of annotations with Mockito tests. 2.1. MockitoJUnitRunnerGoogle Mock can mock non-virtual functions to be used in what we call hi-perf dependency injection. In this case, ... a free function (i.e. a C-style function or a static method). You just need to rewrite your code to use an interface (abstract class). Instead of calling a free function (say ... When you define the mock class using Google Mock ...6 thg 12, 2019 ... Mocking an abstract class is practically just like creating an anonymous class but using convenient tools. It has the same drawbacks and ...Using JMockit to mock autowired interface implementations. We are writing JUnit tests for a class that uses Spring autowiring to inject a dependency which is some instance of an interface. Since the class under test never explicitly instantiates the dependency or has it passed in a constructor, it appears that JMockit doesn't feel …Currently, the unit test that I have uses mocker to mock each class method, including init method. I could use a dependency injection approach, i.e. create an interface for the internal deserializer and proxy interface and add these interfaces to the constructor of the class under test.22 thg 1, 2014 ... aside for the lack of any dependenThis is due to the way mocking is implemented in
Top Travel Destinations in 2024
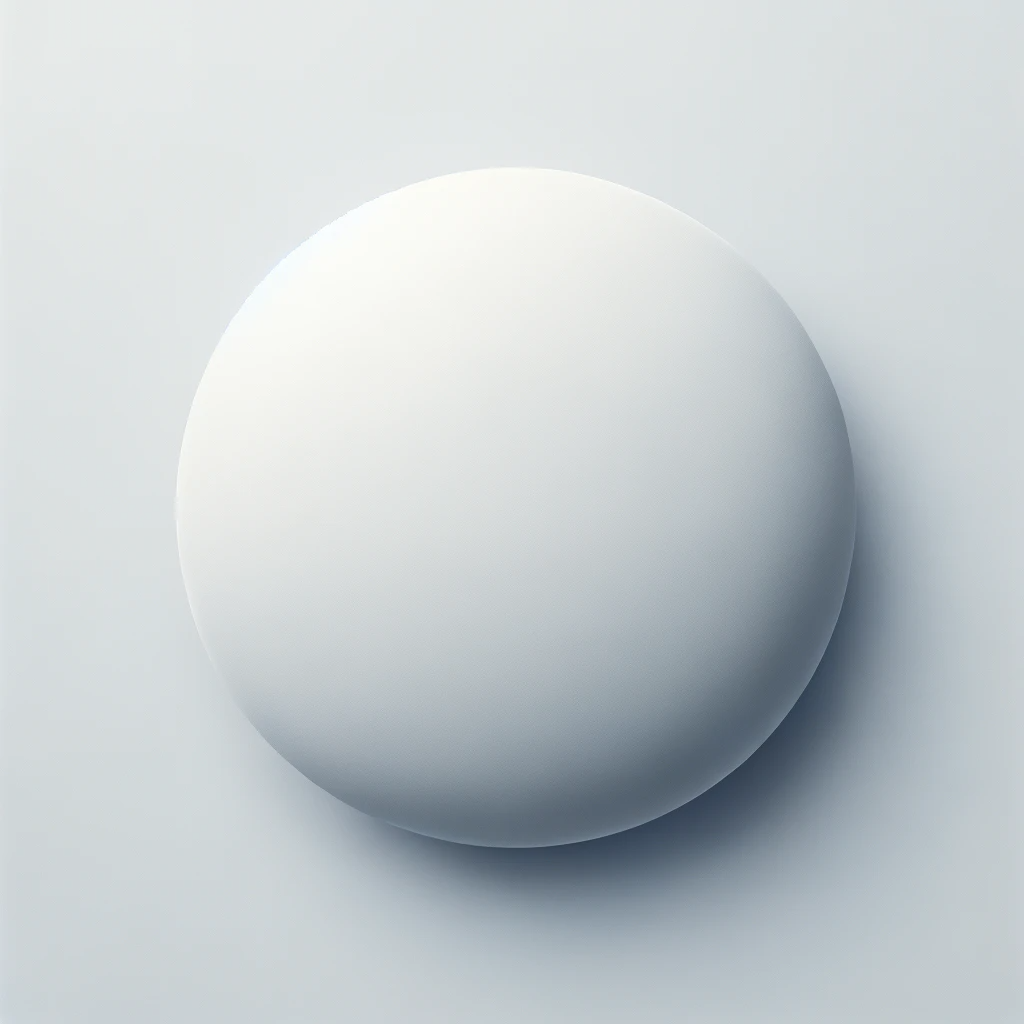
6. I need to mock a call to the findById method of the GenericService. I've this: public class UserServiceImpl extends GenericServiceImpl<User Integer> implements UserService, Serializable { .... // This call i want mock user = findById (user.getId ()); ..... // For example this one calls mockeo well.Dependency injection and class inheritance are not directly related. This means you cannot switch out the base class of your service like this. As I see it you have two ways on how to do this. Option 1: Instead of mocking your BaseApi and providing the mock in your test you need to mock your EntityApi and provide this mock in your test. …Jun 28, 2023 · public abstract class AbstractIndependent { public abstract int abstractFunc(); public String defaultImpl() { return "DEFAULT-1"; } } We want to test the method defaultImpl() , and we have two possible solutions – using a concrete class, or using Mockito. 3. b is a mock, so you shouldn't need to inject anything. After all it isn't executing any real methods (unless you explicitly do so with by calling thenCallRealMethod ), so there is no need to inject any implementation of ClassANeededByClassB. If ClassB is the class under test or a spy, then you need to use the @InjectMocks annotation which ...Currently, the unit test that I have uses mocker to mock each class method, including init method. I could use a dependency injection approach, i.e. create an interface for the internal deserializer and proxy interface and add these interfaces to the constructor of the class under test.Aug 3, 2022 · If there is only one matching mock object, then mockito will inject that into the object. If there is more than one mocked object of the same class, then mock object name is used to inject the dependencies. Mock @InjectMocks Example public abstract class AbstractIndependent { public abstract int abstractFunc(); public String defaultImpl() { return "DEFAULT-1"; } } We want to test the method defaultImpl() , and we have two possible solutions – using a concrete class, or using Mockito.1 Answer. Sorted by: 1. If you want to use a mocked logger in the constructor, you it requires two steps: Create the mock in your test code. Pass it to your production code, e.g. as a constructor parameter. A sample test could look like this:The simplest overloaded variant of the mock method is the one with a single parameter for the class to be mocked: public static <T> T mock(Class<T> …With the hints kindly provided above, here's what I found most useful as someone pretty new to JMockit: JMockit provides the Deencapsulation class to allow you to set the values of private dependent fields (no need to drag the Spring libraries in), and the MockUp class that allows you to explicitly create an implementation of an interface and mock one or more methods of the interface.Aug 3, 2022 · If there is only one matching mock object, then mockito will inject that into the object. If there is more than one mocked object of the same class, then mock object name is used to inject the dependencies. Mock @InjectMocks Example If you can't change your class structure you need to use Mockito.spy instead of Mockito.mock to stub specific method calls but use the real object. public void testCreateDummyRequest () { //create my mock holder Holder mockHolder = new Holder (); MyClass mockObj = Mockito.spy (new MyClass ()); Mockito.doNothing ().when (mockObj).doSomething ...6 thg 12, 2019 ... Mocking an abstract class is practically just like creating an anonymous class but using convenient tools. It has the same drawbacks and ...TL;DR. I am using ReflectionTestUtils#setField() to inject the concrete mapper to the field.. Injecting field. In case I need to test logical flow in the code without the need to use Spring Test Context, I inject few dependencies with Mockito framework.The new method that makes mocking object constructions possible is Mockito.mockConstruction (). This method takes a non-abstract Java class that constructions we're about to mock as a first argument. In the example above, we use an overloaded version of mockConstruction () to pass a MockInitializer as a second argument.Use xUnit and Moq to create a unit test method in C#. Open the file UnitTest1.cs and rename the UnitTest1 class to UnitTestForStaticMethodsDemo. The UnitTest1.cs files would automatically be ...The PHPUnit method getMockForAbstractClass() can be used to generate a partial mock where only the abstract methods of a given class are overridden. The argument list for getMockForAbstractClass() is similar to the argument list for getMock().The big difference is that the list of methods to mock is moved from being the second parameter to being the …For its test, I am looking to inject the mocks as follows but it is not working. The helper comes up as null and I end up having to add a default constructor to be able to throw the URI exception. Please advice a way around this …Mocking is a process where you inject functionality that you don't want to test or an external service, i.e. a service call. Mocking in this scenario makes no sense. You can't mock the base class of the instanciated class, the instanciated class includes the base class and all it's functionality. If the base class called an external service ...Mocks are initialized before each test method. The first solution (with the MockitoAnnotations.initMocks) could be used when you have already configured a specific runner ( SpringJUnit4ClassRunner for example) on your test case. The second solution (with the MockitoJUnitRunner) is the more classic and my favorite. The code is simpler.Starting with version 3.5.0 of Mockito and using the InlineMockMaker, you can now mock object constructions: try (MockedConstruction<A> mocked = mockConstruction (A.class)) { A a = new A (); when (a.check ()).thenReturn ("bar"); } Inside the try-with-resources construct all object constructions are returning a mock. It should never be difficult to write a test for a simple class. However, how to mock static methods is described here PowerMockito mock single static method and return object (thanks to Jorge) how to partially mock a class is already described here: How to mock a call of an inner method from a Junit. I can add following:8. I'm trying to resolve dependency injection with Repository Pattern using Quarkus 1.6.1.Final and OpenJDK 11. I want to achieve Inject with Interface and give them some argument (like @Named or @Qualifier ) for specify the concrete class, but currently I've got UnsatisfiedResolutionException and not sure how to fix it. 1. You're not taking advantage of the spring framework