Rust serialport of Technology
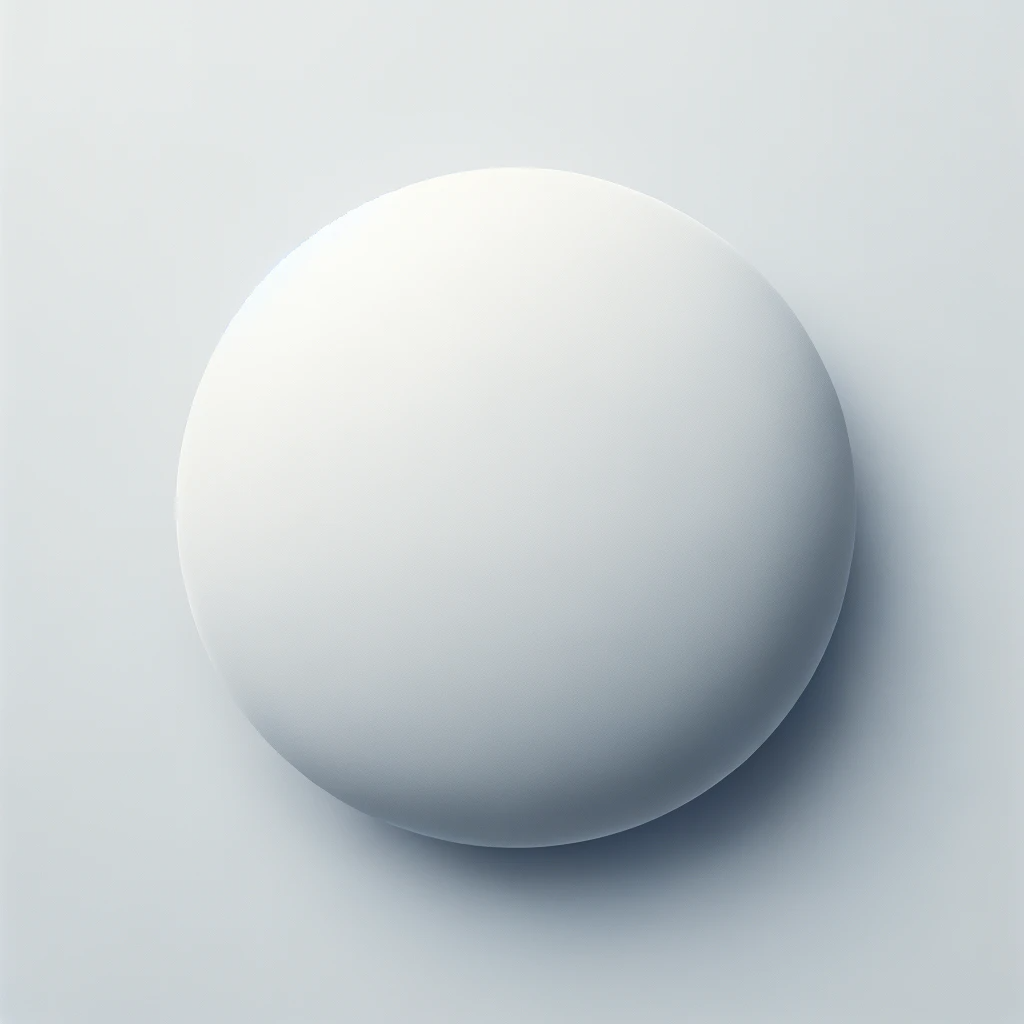
The key property of unions is that all fields of a union share common storage. As a result, writes to one field of a union can overwrite its other fields, and size of a union is determined by the size of its largest field. Union field types are restricted to the following subset of types: Copy types. References ( &T and &mut T for arbitrary T)CDC-ACM USB serial port implementation for usb-device. CDC-ACM is a USB class that's supported out of the box by most operating systems and used for implementing modems and generic serial ports. The SerialPort class implements a stream-like buffered serial port that can be used similarly to a normal UART.A pure Rust Modbus library based on tokio. Modbus is based on a master/slave communication pattern. To avoid confusion with the Tokio terminology the master is called client and the slave is called server in this library. Features. Pure Rust library; Modbus TCP or RTU at your choice; Both async (non-blocking, default) and sync (blocking ...Apr 27, 2019 · We will use the uart_16550 crate to initialize the UART and send data over the serial port. To add it as a dependency, we update our Cargo.toml and main.rs: # in Cargo.toml [dependencies] uart_16550 = "0.2.0" The uart_16550 crate contains a SerialPort struct that represents the UART registers, but we still need to construct an instance of it ... serial-monitor. A serial monitor for USB Serial devices, written in rust. serial-monitor is a command line program which will connect to, and allow you to interact with USB serial devices connected to your host computer. It has been tested on Mac OSX, Linux and Windows. You can use the --list option to display all of the detected USB serial devices, and you can use the --vid, --pid, --port ...The worst offending System.IO.Ports.SerialPort members, ones that not only should not be used but are signs of a deep code smell and the need to rearchitect all IOPSP usage: The DataReceived event (100% redundant, also completely unreliable) The BytesToRead property (completely unreliable)Pro tips: 1) Windows may assign new COM ports to the adapters after every device sleep or reboot. 2) The market leaders in chips for USB to serial are Prolific and FTDI. Both companies are battling knockoffs, and may be blocked in future official Windows drivers. The Linux drivers however work fine with the clones.A data-first Rust-native UI toolkit. Druid is an experimental Rust-native UI toolkit. Its main goal is to offer a polished user experience. There are many factors to this goal, including performance, a rich palette of interactions (hence a widget library to support them), and playing well with the native platform.I'm having difficulty opening opening a serial port using tokio-serial on Win 10. Both the Windows build and the WSL/Debian build. It's a USB serial adapter with an FTDI chip. I can echo characters to it successfully from the Windows command prompt and from the Debian shell in WSL. The code, boiled down, looks like this: pub async fn test(tty_path: &str) -> Result<()> { let settings = tokio ...Finally, an adequate library for wit.ai in Rust. 20 minutes ago escalon-jobs-.1.10 Simple way to scale your jobs application 21 minutes ago mutnet-0.2.0 Unsafe-free and allocation-free, no-std network protocol parsing and in-place manipulation library. ...Serial Port Susurrus/serialport-rs — A cross-platform library that provides access to a serial port; Platform specific. Cross-platform svartalf/rust-battery — Cross-platform information about the notebook batteries ; Linux frol/cgroups-fs — Rust bindings to Linux Control Groups (cgroups) pop-os/dbus-udisks2 — UDisks2 DBus APIThese services require access to the physical serial port (/dev/ttyS0) on the PC. Serial port ls -l: Serial port: crw-rw---- 1 root dialout 4, 64 Jun 13 22:00 /dev/ttyS0. When run as root, the service has no problem communicating via the serial port.1 Answer. As Ice Giant mentions in their answer, you can use static mut to solve this. However, it is unsafe for a good reason: if you use it, you are responsible for making sure that the code is thread safe. It's very easy to misuse and introduce unsound code and undefined behavior into your Rust program, so what I would recommend that you do ...Try to read bytes on the serial port. On success returns the number of bytes read. The function must be called with valid byte array buf of sufficient size to hold the message bytes. If a message is too long to fit in the supplied buffer, excess bytes may be discarded. When there is no pending data, Err(io::ErrorKind::WouldBlock) is returned.Robust Arduino Serial. robust_arduino_serial is a simple and robust serial communication protocol. It was designed to make two arduinos communicate, but can also be useful when you want a computer (e.g. a Raspberry Pi) to communicate with an Arduino. It works with anything that implement the io::Write and io::Read traits.serial2. Serial port communication for Rust. The serial2 crate provides a cross-platform interface to serial ports. It aims to provide a simpler interface than other alternatives. Currently supported features: Simple interface: one SerialPort struct for all supported platforms. List available ports. Custom baud rates on all supported platforms ...For debugging serial device communication issues, use the tee () method of port.readable to split the streams going to or from the serial device. The two streams created can be consumed independently and this allows you to print one to the console for inspection. const [appReadable, devReadable] = port.readable.tee();rust; serial-port; Share. Improve this question. Follow edited Mar 7, 2021 at 23:34. Jason. 4,995 1 1 gold badge 31 31 silver badges 39 39 bronze badges. asked Mar 7, 2021 at 22:57. Marta Marta. 173 1 1 gold badge 2 2 silver badges 11 11 bronze badges. 7.The easiest way to do that with rust would be: cargo install cross cross build --target armv7-unknown-linux-gnueabihf. And with the default setup of cross it will launch a docker container, spawn the build inside that, and cross compile your code. If you want to use podman instead of docker, you need to build cross from master at the moment.Hey, I'm new to Rust/Dioxus and stucked somehow. I would like to write a desktop application to communicate with an external microcontroller via serial port. I can create a serial port and communicate with the microcontroller but don't know how to use it in Dioxus. The serial port is an object stored on the heap and I'm trying to use its pointer in the prop struct to access it when an event ...The Cargo Book. Cargo is the Rust package manager. Cargo downloads your Rust package 's dependencies, compiles your packages, makes distributable packages, and uploads them to crates.io, the Rust community's package registry. You can contribute to this book on GitHub.APIs for Oort, a space fleet programming game. 10 minutes ago. tord-0.1.0. Simple Data structure to store transitive relations. 10 minutes ago. snarkvm-ledger-test-helpers-0.16.6. Test helpers for a decentralized virtual machine. 11 minutes ago. snarkvm-synthesizer-process-0.16.6.To find the paths of available COM ports on the system, you can use the list method. I'd advise omitting any that have an undefined manufacturer property as these normally seem to be things like built-in bluetooth etc. const SerialPort = require ('serialport') SerialPort.list ( (err, ports) => { console.log (ports) })serialport-rs is a general-purpose cross-platform serial port library for Rust. It provides a blocking I/O interface and port enumeration on POSIX and Windows systems. For async I/O functionality, see the mio-serial and tokio-serial crates. Overview. The library exposes cross-platform serial port functionality through the SerialPort trait. This ...1. What you want is non-blocking IO like in the mio crate. For example, if you are using Linux you set up an epoll (event poll) and register one or more files associated with the COM ports you want to watch (something like /dev/ttyS0 ). Then you periodically check the poll for events and act accordingly.3. Left click on "Show Hidden Icons and then right click on the Serial Port Notifier icon. (Image credit: Tom's Hardware) 4. Select the correct COM port and click Rename. (Image credit: Tom's ...It's my first post here. I'm trying to learn Rust programming for embedded devices, and I'd like to set up USB communication for my Nucleo F413ZH board. I've managed to set up everything correctly and I've tested a simple Blink program, which is working. I've tried moving on to USB communication, writing the following two files: main.rs.And traits that extend Ipv4Addr and Ipv6Addr with methods for addition, subtraction, bitwise-and, and bitwise-or operations that are missing in Rust’s standard library. The module only uses stable features so it is guaranteed to compile using the stable toolchain. Organization. IpNet represents an IP network address, either IPv4 or IPv6.Aug 6, 2021 · rust serialport-rs reading blocked. I am trying to use COM RS232 serialport-rs example receive_data and it only works on Mac or and Linux. It does not work on Windows 10. The example is blocked waiting for data and not receiving anything. If I open the COM port with Arduino's serial monitor I see data coming into the PC's port, but Rust's ... Related: serial-core, serial-unix, serial-windows See also: serialport, tokio-serial, mio-serial, uart_16550, btleplug, nvml-wrapper, rppal, acpi, aml, zproto, sgx-isa. Lib.rs is an unofficial list of Rust/Cargo crates, created by kornelski. It contains data from multiple sources, including heuristics, and manually curated data. Content of this ...Mar 7, 2021 · rust; serial-port; Share. Improve this question. Follow edited Mar 7, 2021 at 23:34. Jason. 4,995 1 1 gold badge 31 31 silver badges 39 39 bronze badges. Remember to select exactly the same serial port you have identified in steps above. Setting Serial Communication in PuTTY on Windows Setting Serial Communication in PuTTY on Linux Then open serial port in terminal and check, if you see any log printed out by ESP32-C3. The log contents depend on application loaded to ESP32-C3, see Example Output ...It's my first post here. I'm trying to learn Rust programming for embedded devices, and I'd like to set up USB communication for my Nucleo F413ZH board. I've managed to set up everything correctly and I've tested a simple Blink program, which is working. I've tried moving on to USB communication, writing the following two files: main.rs.Hello world ESP32-S3 with Rust8 Answers. import serial.tools.list_ports ports = list (serial.tools.list_ports.comports ()) for p in ports: print p. Call QueryDosDevice with a NULL lpDeviceName to list all DOS devices. Then use CreateFile and GetCommConfig with each device name in turn to figure out whether it's a serial port.serialport-rs is a general-purpose cross-platform serial port library for Rust. It provides a blocking I/O interface and port enumeration on POSIX and Windows systems. For async I/O functionality, see the mio-serial and tokio-serial crates. Overview. The library exposes cross-platform serial port functionality through the SerialPort trait. This ...Ok, while skimming at your post, I saw: static mut GRID: Option<Box<dyn Grid>> = None; which automatically triggered my writing this: static mut is a very very unsafe feature (much more than most of us would imagine), because of immutability guarantees Rust uses whenever it sees a shared reference (i.e., whenever you unsafe { &GRID } it is Undefined Behavior to ever unsafe { &mut GRID ...Jot down the listening port for serial port 0. NetBurner embedded device TCP configuration page. Back in the Virtual COM Port application, click on "Add" in the Virtual Serial Port dialog box, which will bring up the "Edit Connection" window. Virtual COMM Port application screenshot. Next, from the "Select serial port" drop-down ...Rust でシリアル通信を扱う. いちばん下へジャンプ. Hideaki Tai 2021/12/24. デファクトスタンダード的なやつ (使いやすそう) Bryant / serialport-rs · GitLab. 返信. Hideaki Tai 2021/12/24. tokio-rs の mio で動くようにした serialport. GitHub - berkowski/mio-serial.parking_lot. Documentation (synchronization primitives) Documentation (core parking lot API) Documentation (type-safe lock API) This library provides implementations of Mutex, RwLock, Condvar and Once that are smaller, faster and more flexible than those in the Rust standard library, as well as a ReentrantMutex type which supports recursive locking. It also exposes a low-level API for creating ...Mio is a fast, low-level I/O library for Rust focusing on non-blocking APIs and event notification for building high performance I/O apps with as little overhead as possible over the OS abstractions. API documentation. v0.8; v0.7; This is a low level library, if you are looking for something easier to get started with, see Tokio. UsageArduino ESP32 Serial2 loopback. The working is simple, we write something in arduino serial console and program will echo it. The serial console is connected to Serial (UART0), which is also used for loading arduino code to ESP32. The program will be monitoring UART0, and if it sees any data on UART0 it will write that data to UART2.1 Answer. Sorted by: 8. Apparently, the serialport crate that I was using requires you to set the command. port.write_data_terminal_ready (true); in order for it to start reading data. On Linux this works perfectly fine without it. Rip 4 hours trying to change what IO reader I was using.A cross-platform serial port library in Rust. Provides a blocking I/O interface and port enumeration including USB device information. Note: This is a fork of the original serialport-rs project on GitLab. Please note there have been some changes to both the supported targets and which3. Left click on “Show Hidden Icons and then right click on the Serial Port Notifier icon. (Image credit: Tom's Hardware) 4. Select the correct COM port and click Rename. (Image credit: Tom's ...We count on your support to pay the people develop Node Serialport and work on the ecosystem projects that further our goals. In addition to buying test equipment and hiring contractors as needed. Our two funding goals; Hosting and Hardware: This covers our test, build and deployment infrastructure costs, and buying test hardware.serialport-rs Public. A cross-platform serial port library in Rust. Provides a blocking I/O interface and port enumeration including USB device information. Rust 254 69 38 (1 issue needs help) 11 Updated 2 weeks ago. electron-serialport Public. An example of how to use serialport in an electron app. {"payload":{"allShortcutsEnabFeb 19, 2021 · I am developing my Rust projecIt's an asynchronous communication protocol where two de