Solve a system of equations matlab of Technology
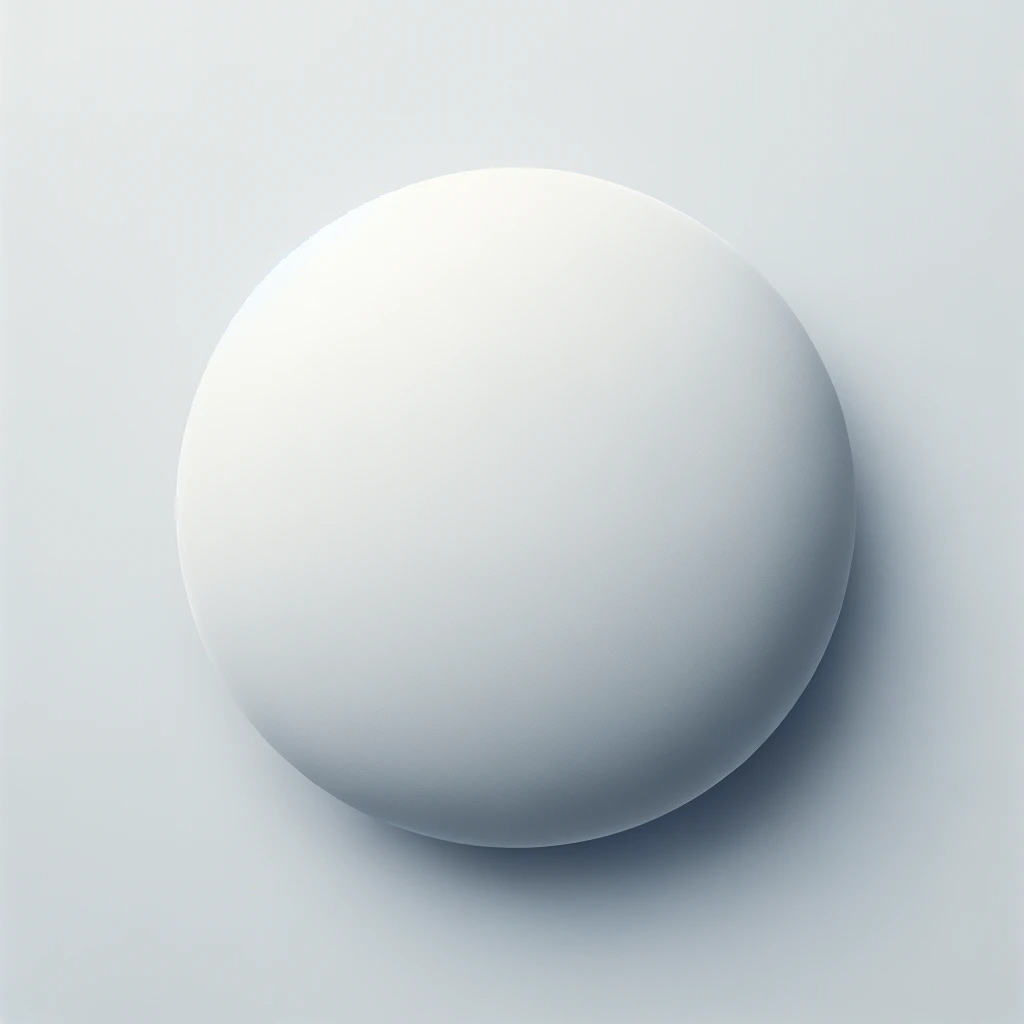
1 Answer. You can use multiple calls of solve to get solutions for x1 and x2. In this problem you can solve the first equation for x1, and then plug that into the second equation to get x2 in terms of x3, x4, and x5. You can then substitute the new value of x2 back into your solution of x1. The subs function is used to substitute the solved ...2. Certainly, you should have a look at your function yprime. Using some simple model that shares the number of differential state variables with your problem, have a look at this example. function dyds = yprime (s, y) dyds = zeros (2, 1); dyds (1) = y (1) + y (2); dyds (2) = 0.5 * y (1); end. yprime must return a column vector that holds the ...All MATLAB ® ODE solvers can solve systems of equations of the form y ' = f (t, y), or problems that involve a mass matrix, M (t, y) y ' = f (t, y). The solvers all use similar syntaxes. The ode23s solver only can solve problems with a …Create a vector of ones for the right-hand side of the linear equation Ax = b. The number of rows in A and b must be equal. b = ones (size (A,2),1); Solve the linear system Ax = b using mldivide and time the calculation. tic x1 = A\b; t1 = toc. t1 = 0.0514. Now, solve the system again using linsolve. Jul 28, 2020 · At first, you need to write your 12 coupled ODEs. Make sure that are in first order form, if not convert them. Next, define your variables. You can import the data in Matlab from your excel sheet. Finally, call the Euler's method function (for example, shown in this tutorial) to solve the coupled equations. Solve the system of equations starting at the point [0,0]. fun = @root2d; x0 = [0,0]; x = fsolve(fun,x0) Equation solved. fsolve completed because the vector of function values is near zero as measured by the value of the function tolerance, and the problem appears regular as measured by the gradient. ... You must have a MATLAB Coder license to ...Solve a linear system with both mldivide and linsolve to compare performance. mldivide is the recommended way to solve most linear systems of equations in MATLAB®. …Script 2 Save C Reset D MATLAB Documentation 1 Create the coefficient matrix. Store the coefficient matrix in A. 3 Create the column matrix of constants. Store ...To solve the Lotka-Volterra equations in MATLAB®, write a function that encodes the equations, specify a time interval for the integration, and specify the initial conditions. Then you can use one of the ODE solvers, such as ode45 , to simulate the system over time.For a comparison of numeric and symbolic solvers, see Select Numeric or Symbolic Solver. An equation or a system of equations can have multiple solutions. To find these solutions numerically, use the function vpasolve. For polynomial equations, vpasolve returns all solutions. For nonpolynomial equations, vpasolve returns the first solution it ... Let us see how to solve a system of linear equations in MATLAB. Here are the various operators that we will be deploying to execute our task : \ operator : A \ B is the matrix division of A into B, which is roughly the same as INV(A) * B.If A is an NXN matrix and B is a column vector with N components or a matrix with several such columns, then X = …Apr 6, 2012 · How can i solve a system of nonlinear differential equations using Matlab?? here is an example of what i'm talking about it's not the problem that i'm working in but it had the same form. //// x'=3x+y//// y'=y-x+y^4+z^4//// z'=y+z^4+y^4+3/// the ' means the derivative. i'll appreciate your help, best regards! To solve this equation in MATLAB®, you need to code the equation, the initial conditions, and the boundary conditions, then select a suitable solution mesh before calling the solver pdepe. You either can include the required functions as local functions at the end of a file (as done here), or save them as separate, named files in a directory ...The first 3 equations must therefore be purely numeric, in which case you are asking solve() to solve for three numeric variables being equal to 0 and have all the symbolic information in the remaining 3 equations.This tells us that the only solution is x = -2, y = 5, z = -6. Method 2: Using left division. The motivation for this method is complicated. The algorithm is Gaussian elimination, which is not actually a division, but that a division symbol is used by MATLAB to apply this algorithm, as shown below.@Christopher Van Horn I can assure you that the vast majority of people posting questions have not bothered to look for the solution in the forum or elsewhere as evidenced by dozens of questions asked every day that have 20+ or 100+ identical solutions in the forum. Too many people want to be given a solution with their exact variable …All MATLAB ® ODE solvers can solve systems of equations of the form y ' = f (t, y), or problems that involve a mass matrix, M (t, y) y ' = f (t, y). The solvers all use similar syntaxes. The ode23s solver only can solve problems with a mass matrix if the mass matrix is constant. The solve function returns a structure when you specify a single output argument and multiple outputs exist. Solve a system of equations to return the solutions in a structure array. syms u v eqns = [2*u + v == 0, u - v == 1]; S = solve (eqns, [u v]) S = struct with fields: u: 1/3 v: -2/3.When A is a large sparse matrix, you can solve the linear system using iterative methods, which enable you to trade-off between the run time of the calculation and the precision of the solution. This topic describes the iterative methods available in MATLAB ® to solve the equation A*x = b. Direct vs. Iterative MethodsSolve the system using the dsolve function which returns the solutions as elements of a structure. S = dsolve (odes) S = struct with fields: v: C1*cos (4*t)*exp (3*t) - C2*sin …Description example x = A\B solves the system of linear equations A*x = B. The matrices A and B must have the same number of rows. MATLAB ® displays a warning message if A is badly scaled or nearly singular, but performs the calculation regardless. If A is a scalar, then A\B is equivalent to A.\B.1. Ok, turns out it was just a minor mistake where the x-variable was not defined as a function of y (as x' (t)=y according to the problem. So: Below is a concrete example on how to solve a differential equation system using Runge Kutta 4 in matlab:You can't just "solve" such a problem, because infinitely many solutions may exist. You will need to pick exactly one more variable to remain fixed. For example: sol = vpasolve (eqn1, eqn2,eqn3,eqn4,eqn5,eqn6,eqn7,eqn8) To learn MATLAB, try the doc. There's a nice Getting Started section for every part of MATLAB.From a numerical standpoint, a more efficient way to solve this system of equations is with x0 = A\b, which (for a rectangular matrix A) calculates the least-squares solution. In that case, you can check the accuracy of the solution with norm(A*x0-b)/norm(b) and the uniqueness of the solution by checking if rank(A) is equal to the number of ...... system as a MATLAB function f = @(t,x) [-x(1)+3*x(3);-x(2)+2*x(3);x(1)^2-2*x(3)];. The numerical solution on the interval $[0,1.5]$ with $x(0)=0,y(0)=1/2 isTo solve the Lotka-Volterra equations in MATLAB®, write a function that encodes the equations, specify a time interval for the integration, and specify the initial conditions. Then you can use one of the ODE solvers, such as ode45 , to simulate the system over time. Visualize the system of equations using fimplicit.To set the x-axis and y-axis values in terms of pi, get the axes handles using axes in a.Create the symbolic array S of the values -2*pi to 2*pi at intervals of pi/2.To set the ticks to S, use the XTick and YTick properties of a.To set the labels for the x-and y-axes, convert S to character vectors. Use arrayfun to …Here is a modified version to match your notation of an old implementation of mine for Newton's method, and this could be easily vectorized for a multi-dimensional nonlinear equation system using varargin input, and do a string size check on the inline function you passed to the following function.As you noted, the analyticalInverseKinematics solver is presently limited to 6-DoF robots with a wrist. Other solutions (general 6-DoF solutions and solutions for robots with less than 6 DoF) are certainly possible, and we are looking to include those in future enhancements.Description. Nonlinear system solver. Solves a problem specified by. F ( x) = 0. for x, where F ( x ) is a function that returns a vector value. x is a vector or a matrix; see Matrix Arguments. example. x = fsolve (fun,x0) starts at …Visualize the system of equations using fimplicit.To set the x-axis and y-axis values in terms of pi, get the axes handles using axes in a.Create the symbolic array S of the values -2*pi to 2*pi at intervals of pi/2.To set the ticks to S, use the XTick and YTick properties of a.To set the labels for the x-and y-axes, convert S to character vectors. Use arrayfun to …1 Answer. When you use the SOLVE function (from the Symbolic Toolbox) you can specify the variables you want to solve for. For example, let's say you have three equations with variables x, y, and z and constants a and b. The following will give you a structure S with fields 'x', 'y', and 'z' containing symbolic equations for those variables ...Create a vector of ones for the right-hand side of the linear equation Ax = b. The number of rows in A and b must be equal. b = ones (size (A,2),1); Solve the linear system Ax = b using mldivide and time the calculation. tic x1 = A\b; t1 = toc. t1 = 0.0514. Now, solve the system again using linsolve.MATLAB backslash operator is used to solving a linear equation of the form a*x = b, where ‘a’ and ‘b’ are matrices and ‘x’ is a vector. The solution of this equation is given by x = a \ b, but it works only if the number of rows in ‘a’ and ‘b’ is equal. If the number of rows is not equal, and ‘a’ is not a scalar, we will ...Tridiagonal Matrix Convention. For these implementations, I use the following convention for denoting the elements of the tridiagonal matrix : Most other references have 's ranging from to both in the definition of the tridiagonal matrix and in the algorithm used to solve the corresponding linear system. In this implementation, I have the 's ...All MATLAB ® ODE solvers can solve systems of equations of the form y ' = f (t, y), or problems that involve a mass matrix, M (t, y) y ' = f (t, y). The solvers all use similar syntaxes. The ode23s solver only can solve problems with a mass matrix if the mass matrix is constant. Apr 6, 2012 · How can i solve a system of nonlinear differential equations using Matlab?? here is an example of what i'm talking about it's not the problem that i'm working in but it had the same form. //// x'=3x+y//// y'=y-x+y^4+z^4//// z'=y+z^4+y^4+3/// the ' means the derivative. i'll appreciate your help, best regards! Solving trigonometric equation using... Learn more about trigonometry, solve, trigonometric equation MATLABTo solve for the desired variables, simply list them as per the documentation: s = solve (b,q1,q2,q3,q4) or. [q1,q2,q3,q4] = solve (b,q1,q2,q3,q4) Now you will obtain non-zero solutions. However, you'll still get a warning as you obviously have three equations and are trying to solve for four unknowns and there are possibly an infinite number ...When solving for multiple variables, it can be more convenient to store the outputs in a structure array than in separate variables. The solve function returns a structure when you specify a single output argument and multiple outputs exist. Solve a system of equations to return the solutions in a structure array.x = A\B solves the system of linear equations A*x = B. The matrices A and B must have the same number of rows. MATLAB ® displays a warning message if A is badly scaled or nearly singular, but performs the calculation regardless. If A is a square n -by- n matrix and B is a matrix with n rows, then x = A\B is a solution to the equation A*x = B ...x = A\B solves the system of linear equations A*x = B. The matrices A and B must have the same number of rows. MATLAB ® displays a warning message if A is badly scaled or nearly singular, but performs the calculation regardless. If A is a square n -by- n matrix and B is a matrix with n rows, then x = A\B is a solution to the equation A*x = B ...x = symmlq(A,b) attempts to solve the system of linear equations A*x = b for x using the Symmetric LQ Method.When the attempt is successful, symmlq displays a message to confirm convergence. If symmlq fails to converge after the maximum number of iterations or halts for any reason, it displays a diagnostic message that includes the relative residual …27 Mar 2020 ... sense = '='; m.quadcon(i).name = sprintf('qcon%d', i); end % Add variable names vnames = cell(n,1); for i=1:n vnames{i} = sprintf('x%d', i); end ...For a comparison of numeric and symbolic solvers, see Select Numeric or Symbolic Solver. An equation or a system of equations can have multiple solutions. To find these solutions numerically, use the function vpasolve. For polynomial equations, vpasolve returns all solutions. For nonpolynomial equations, vpasolve returns the first solution it ... x = A\B solves the system of linear equations A*x = B. The matrices A and B must have the same number of rows. MATLAB ® displays a warning message if A is badly scaled or nearly singular, but performs the calculation regardless. If A is a square n -by- n matrix and B is a matrix with n rows, then x = A\B is a solution to the equation A*x = B ...Reduced Row Echelon Form of a matrix is used to find the rank of a matrix and further allows to solve a system of linear equations. A matrix is in Row Echelon form if. All rows consisting of only zeroes are at the bottom. The first nonzero element of a nonzero row is always strictly to the right of the first nonzero element of the row above it.When solving for multiple variables, it can be more convenient to store the outputs in a structure array than in separate variables. The solve function returns a structure when you specify a single output argument and multiple outputs exist. Solve a system of equations to return the solutions in a structure array.x = A\B solves the system of linear equations A*x = B. The matrices A and B must have the same number of rows. MATLAB ® displays a warning message if A is badly scaled or nearly singular, but performs the calculation regardless. If A is a square n -by- n matrix and B is a matrix with n rows, then x = A\B is a solution to the equation A*x = B ...The inputs to solve are a vector of equations, and a vector of variables to solve the equations for. sol = solve ( [eqn1, eqn2, eqn3], [x, y, z]); xSol = sol.x ySol = sol.y zSol = sol.z. xSol = 3 ySol = 1 zSol = -5. solve returns the solutions in a structure array. To access the solutions, index into the array. Suppose you have the system. x 2 y 2 = 0 x - y 2 = α , and yFrom a numerical standpoint, a more efficient way to sAt first, you need to write your 12 coupled ODE